|
|
|
Perl
Variables
Before you can proceed much further with Perl, you'll need
some understanding of variables. A variable is just a place to
store a value, so you can refer to it or manipulate it
throughout your program. Perl has three types of variables:
scalars, arrays, and hashes.
A scalar variable stores a single (scalar) value. Perl scalar
names are prefixed with a dollar sign ($), so for example, $x,
$y, $z, $username, and $url are all examples of scalar
variable names. Here's how variables are used:
CODE |
$foo = 1;
$name = "Fred";
$pi = 3.141592;
|
You do not need to declare a variable before using it; just
drop it into your code. A scalar can hold data of any type, be
it a string, a number, or whatnot. You can also drop scalars
into double-quoted strings:
CODE |
$fnord = 23;
$blee = "The magic number is $fnord.";
|
Now if you print $blee, you will get "The magic number is
23."
Let's edit first.pl again and add some scalars to it:
CODE |
#!/usr/bin/perl
$classname = "CGI Programming 101";
print "Hello there. What is your name?n";
$you = <STDIN>;
chomp($you);
print "Hello, $you. Welcome to $classname.n";
|
Save, and run the script in the Unix shell. (This program will
not work as a CGI.) This time, the program will prompt you for
your name, and read your name using the following line:
STDIN is standard input. This is the default input channel for
your script; if you're running your script in the shell, STDIN
is whatever you type as the script runs.
The program will print "Hello there. What is your
name?", then pause and wait for you to type something in.
(Be sure to hit return when you're through typing your name.)
Whatever you typed is stored in the scalar variable $you.
Since $you also contains the carriage return itself, we use
to remove the carriage return from the end of the string you
typed in. The following print statement:
CODE |
print "Hello, $you. Welcome to $classname.n";
|
substitutes the value of $you that you entered. The
"n" at the end if the line is the perl syntax for a
carriage return.
Arrays
An array stores a list of values. While a scalar variable can
only store one value, an array can store many. Perl array
names are prefixed with an at-sign (@). Here is an example:
CODE |
@colors = ("red","green","blue");
|
In Perl, array indices start with 0, so to refer to the first
element of the array @colors, you use $colors[0]. Note that
when you're referring to a single element of an array, you
prefix the name with a $ instead of the @. The $-sign again
indicates that it's a single (scalar) value; the @-sign means
you're talking about the entire array.
If you wanted to loop through an array, printing out all of
the values, you could print each element one at a time:
CODE |
#!/usr/bin/perl
# this is a comment
# any line that starts with a "#" is a
comment.
@colors = ("red","green","blue");
print "$colors[0]n";
print "$colors[1]n";
print "$colors[2]n";
|
Or, a much easier way to do this is to use the foreach
construct:
CODE |
#!/usr/bin/perl
# this is a comment
# any line that starts with a "#" is a
comment.
@colors = ("red","green","blue");
foreach $i (@colors) {
print "$in";
}
|
For each iteration of the foreach loop, Perl sets $i to an
element of the @colors array - the first iteration, $i is
"red". The braces {} define where the loop begins
and end, so for any code appearing between the braces, $i is
set to the current loop iterator.
Array Functions
Since an array is an ordered list of elements, there are a
number of functions you can use to get data out of (or put
data into) the list:
CODE |
@colors = ("red","green","blue","cyan","magenta","black","yellow");
$elt = pop(@colors); # returns "yellow", the
last value
# of the array.
$elt = shift(@colors); # returns "red", the
first value
# of the array.
|
In these examples we've set $elt to the value returned, but
you don't have to do that - if you just wanted to get rid of
the first value in an array, for example, you'd just shift(@arrayname).
Both shift and pop affect the array itself, by removing an
element; in the above example, after you pop
"yellow" off the end of @colors, the array is then
equal to ("red", "green",
"blue", "cyan", "magenta",
"black"). And after you shift "red" off
the front, the array becomes ("green",
"blue", "cyan", "magenta",
"black").
You can also add data to an array:
CODE |
@colors = ("green", "blue",
"cyan", "magenta",
"black");
push(@colors,"orange"); # adds
"orange" to the end of the
# @colors array
@colors now becomes ("green",
"blue", "cyan",
"magenta", "black",
"orange").
@morecolors = ("purple","teal","azure");
push(@colors,@morecolors); # appends the values in @morecolors
# to the end of @colors
@colors now becomes ("green",
"blue", "cyan",
"magenta", "black",
"orange", "purple", "teal","azure").
Here are a few other useful functions for array
manipulation:
@colors = ("green", "blue",
"cyan", "magenta",
"black");
sort(@colors) # sorts the values of @colors
# alphabetically
@colors now becomes ("black",
"blue", "cyan", "green",
"magenta" ). Note that sort does not change
the actual values of the array itself, so if you want
to save the sorted array, you have to do something
like this:
@sortedlist = sort(@colors);
The same thing is true for the reverse function:
@colors = ("green", "blue",
"cyan", "magenta",
"black");
reverse(@colors) # inverts the @colors array
@colors now becomes ("black",
"magenta", "cyan",
"blue", "green" ). Again, if you
want to save the inverted list, you must assign it to
another array.
$#colors # length-1 of the @colors array, or
# the last index of the array
|
In this example, $#colors is 4. The actual length of the array
is 5, but since Perl lists count from 0, the index of the last
element is length - 1. If you want the actual length of the
array (the number of elements), you'd use the scalar function:
CODE |
scalar(@colors); # the actual length of the array
In this case, scalar(@colors) is equal to 5.
join(", ",@colors) # joins @colors into a
single
# string separated by the
# expression ", "
|
@colors becomes a single string: "black, magenta, cyan,
blue, green".
Hashes
A hash is a special kind of array - an associative array, or
paired group of elements. Perl hash names are prefixed with a
percent sign (%), and consist of pairs of elements - a key and
a data value. Here's how to define a hash:
Hash Name key value
CODE |
%pages = ( "fred", "http://www.blah.com/~fred/",
"beth", "http://www.blah.com/~beth/",
"john", "http://www.blah.com/~john/"
);
|
Another way to define a hash would be as follows:
CODE |
%pages = ( fred => "http://www.blah.com/~fred/",
beth => "http://www.blah.com/~beth/",
john => "http://www.blah.com/~john/" );
|
The => operator is a synonym for ", ". It also
automatically quotes the left side of the argument, so
enclosing quotes are not needed.
This hash consists of a person's name for the key, and their
URL as the data element. You refer to the individual elements
of the hash with a $ sign (just like you did with arrays),
like so:
In this case, "fred" is the key, and $pages{'fred'}
is the value associated with that key - in this case, it would
be "http://www.blah.com/~fred/".
If you want to print out all the values in a hash, you'll need
a foreach loop, like follows:
CODE |
foreach $key (keys %pages) {
print "$key's page: $pages{$key}n";
}
|
This example uses the keys function, which returns an array
consisting only of the keys of the named hash. One drawback is
that keys %hashname will return the keys in random order - in
this example, keys %pages could return ("fred",
"beth", "john") or ("beth",
"fred", "john") or any combination of the
three. If you want to print out the hash in exact order, you
have to specify the keys in the foreach loop:
CODE |
foreach $key ("fred","beth","john")
{
print "$key's page: $pages{$key}n";
}
|
Hashes will be especially useful when you use CGIs that parse
form data, because you'll be able to do things like $FORM{'lastname'}
to refer to the "lastname" input field of your form.
Let's write a simple CGI using the above hash, to create a
page of links:
CODE |
#!/usr/bin/perl
#
# the # sign is a comment in Perl
%pages = ( "fred" => "http://www.blah.com/~fred/",
"beth" => "http://www.blah.com/~beth/",
"john" => "http://www.blah.com/~john/"
);
print "Content-type:text/htmlnn";
print <<EndHdr;
<html><head><title>URL
List</title></head>
<body bgcolor="#ffffff">
<p>
<h2>URL List</h2>
<ul>
EndHdr
foreach $key (keys %pages) {
print "<li><a href=\"$pages{$key}\">$key</a>n";
}
print <<EndFooter;
</ul>
<p>
</body>
</html>
EndFooter
|
Call it urllist.cgi, save it, and change the permissions so
it's executable. Then call it up in your web browser. You
should get a page listing each person's name, hotlinked to
their actual URL.
Chapter 3 will expand this concept as we look at environment
variables and the GET method of posting forms.
Hash Functions
Here is a quick overview of the Perl functions you can use
when working with hashes.
CODE |
delete $hash{$key} # deletes the specified key/value
pair,
# and returns the deleted value
exists $hash{$key} # returns true if the specified key
exists
# in the hash.
keys %hash # returns a list of keys for that hash
values %hash # returns a list of values for that hash
scalar %hash # returns true if the hash has elements
# defined (e.g. it's not an empty hash)
|
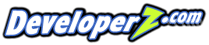
|
|