|
|
|
Error
Handling
This script will enable you to change the way php handles your
error messages. You will be able to send error details via
email and redirect the visitor to an error page! It makes use
of the PHP4 feature set_error_handler. You need to have mail()
properly configured on your system.
Note: This code has to be at the very top of your php file!
CODE |
<?
// Decide what errors to report:
error_reporting (E_ERROR | E_WARNING | E_NOTICE);
// Webmaster email and error page url:
$Wmemail = 'you@yoursite.com';
$ErrorPage = 'http://www.yoursite.com/error.html';
// This function will check the error type:
function MailErrorHandler($errno, $errstr, $errfile='?',
$errline= '?')
{
global $Wmemail, $ErrorPage;
if (($errno & error_reporting()) == 0)
return;
$err = '';
switch($errno) {
case E_ERROR: $err = 'FATAL'; break;
case E_WARNING: $err = 'ERROR'; break;
case E_NOTICE: return;
}
// Send the error details via email:
mail($Wmemail,
"PHP: $errfile, $errline",
"$errfile, Line $errlinen$err($errno)n$errstr");
// Redirect to the error page:
print '<META HTTP-EQUIV="Refresh"
CONTENT="0;url='.$ErrorPage.'">';
die();
}
set_error_handler('MailErrorHandler');
?>
|
You can generate an error for testing by using an undefined
variable as a function call:
Here you have the error levels and description:
CODE |
E_NOTICE
Notices are not printed by default, and indicate that
the script encountered something that could indicate
an error, but could also happen in the normal course
of running a script. For example, trying to access the
value of a variable which has not been set, or calling
stat() on a file that doesn't exist.
E_WARNING
Warnings are printed by default, but do not interrupt
script execution. These indicate a problem that should
have been trapped by the script before the call was
made. For example, calling ereg() with an invalid
regular expression.
E_ERROR
Errors are also printed by default, and execution of
the script is halted after the function returns. These
indicate errors that can not be recovered from, such
as a memory allocation problem.
E_PARSE
Parse errors should only be generated by the parser.
The code is listed here only for the sake of
completeness.
E_CORE_ERROR
This is like an E_ERROR, except it is generated by the
core of PHP. Functions should not generate this type
of error.
E_CORE_WARNING
This is like an E_WARNING, except it is generated by
the core of PHP. Functions should not generate this
type of error.
E_COMPILE_ERROR
This is like an E_ERROR, except it is generated by the
Zend Scripting Engine. Functions should not generate
this type of error.
E_COMPILE_WARNING
This is like an E_WARNING, except it is generated by
the Zend Scripting Engine. Functions should not
generate this type of error.
E_USER_ERROR
This is like an E_ERROR, except it is generated in PHP
code by using the PHP function trigger_error().
Functions should not generate this type of error.
E_USER_WARNING
This is like an E_WARNING, except it is generated by
using the PHP function trigger_error(). Functions
should not generate this type of error.
E_USER_NOTICE
This is like an E_NOTICE, except it is generated by
using the PHP function trigger_error(). Functions
should not generate this type of error.
E_ALL
All of the above. Using this error_reporting level
will show all error types.
|
Tutorial By Xtasy
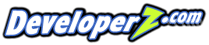
|
|